How to develop Scalable Programs as a Developer By Gustavo Woltmann
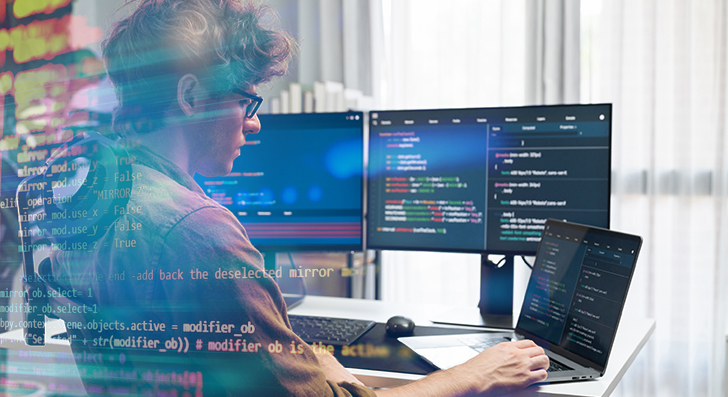
Scalability usually means your application can handle advancement—additional end users, much more details, plus more website traffic—with no breaking. As being a developer, building with scalability in your mind saves time and strain later on. Listed here’s a transparent and functional manual to help you start out by Gustavo Woltmann.
Design and style for Scalability from the beginning
Scalability isn't really something you bolt on afterwards—it should be section of the plan from the beginning. Lots of programs are unsuccessful after they mature speedy since the first style can’t cope with the extra load. Being a developer, you need to Consider early regarding how your technique will behave stressed.
Commence by coming up with your architecture to become versatile. Stay clear of monolithic codebases exactly where anything is tightly related. As an alternative, use modular style or microservices. These designs crack your application into smaller sized, impartial sections. Each module or support can scale By itself without the need of impacting The full procedure.
Also, consider your database from working day just one. Will it have to have to handle a million end users or just a hundred? Choose the proper form—relational or NoSQL—dependant on how your data will develop. Program for sharding, indexing, and backups early, Even though you don’t need to have them yet.
An additional crucial position is to stop hardcoding assumptions. Don’t produce code that only will work less than present-day conditions. Think about what would come about When your consumer base doubled tomorrow. Would your app crash? Would the database slow down?
Use design styles that aid scaling, like information queues or event-pushed units. These assistance your application cope with far more requests with no receiving overloaded.
If you Construct with scalability in your mind, you're not just preparing for fulfillment—you might be cutting down foreseeable future head aches. A nicely-planned procedure is simpler to take care of, adapt, and mature. It’s superior to arrange early than to rebuild later.
Use the ideal Databases
Selecting the correct database can be a essential A part of setting up scalable purposes. Not all databases are built a similar, and using the wrong you can slow you down or perhaps lead to failures as your app grows.
Start by being familiar with your knowledge. Is it highly structured, like rows in a very table? If Of course, a relational database like PostgreSQL or MySQL is a good healthy. These are generally powerful with interactions, transactions, and consistency. In addition they assist scaling methods like read replicas, indexing, and partitioning to take care of a lot more traffic and knowledge.
In case your facts is more adaptable—like user action logs, item catalogs, or files—take into account a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at handling huge volumes of unstructured or semi-structured details and may scale horizontally additional effortlessly.
Also, think about your examine and publish styles. Have you been accomplishing plenty of reads with less writes? Use caching and skim replicas. Are you currently dealing with a major write load? Look into databases that will cope with high compose throughput, or maybe party-based info storage devices like Apache Kafka (for non permanent data streams).
It’s also wise to think ahead. You may not want Innovative scaling options now, but deciding on a database that supports them indicates you won’t want to change later on.
Use indexing to hurry up queries. Stay clear of unnecessary joins. Normalize or denormalize your information according to your accessibility designs. And often keep an eye on databases functionality while you improve.
In short, the proper database depends on your app’s composition, velocity requires, And exactly how you be expecting it to improve. Acquire time to choose sensibly—it’ll help you save many issues later on.
Enhance Code and Queries
Quick code is key to scalability. As your application grows, just about every smaller hold off adds up. Badly composed code or unoptimized queries can slow down performance and overload your procedure. That’s why it’s imperative that you Make productive logic from the start.
Start by crafting cleanse, straightforward code. Steer clear of repeating logic and remove something avoidable. Don’t select the most sophisticated solution if a straightforward one particular functions. Keep the features brief, concentrated, and straightforward to test. Use profiling tools to search out bottlenecks—areas where your code can take also long to operate or utilizes far too much memory.
Following, look at your database queries. These typically slow factors down greater than the code alone. Ensure Each individual query only asks for the info you actually will need. Steer clear of Pick out *, which fetches every thing, and rather pick out particular fields. Use indexes to hurry up lookups. And stay clear of carrying out a lot of joins, especially across significant tables.
Should you detect exactly the same knowledge getting asked for again and again, use caching. Retailer the final results temporarily making use of instruments like Redis or Memcached so you don’t must repeat high-priced functions.
Also, batch your databases operations once you can. Instead of updating a row one by one, update them in groups. This cuts down on overhead and can make your application extra efficient.
Remember to examination with substantial datasets. Code and queries that do the job fine with 100 information may possibly crash when they have to deal with 1 million.
In a nutshell, scalable apps are rapid applications. Keep the code limited, your queries lean, and use caching when desired. These ways help your application stay smooth and responsive, whilst the load will increase.
Leverage Load Balancing and Caching
As your application grows, it's to deal with far more end users plus much more website traffic. If all the things goes as a result of a person server, it will eventually immediately turn into a bottleneck. That’s wherever load balancing and caching come in. Both of these applications assistance keep the application quickly, stable, and scalable.
Load balancing spreads incoming visitors across various servers. In lieu of a person server executing the many operate, the load balancer routes consumers to distinct servers according to availability. This means no one server will get overloaded. If a single server goes down, the load balancer can send visitors to the Some others. Equipment like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this very easy to put in place.
Caching is about storing information briefly so it can be reused promptly. When end users request a similar data once more—like an item website page or perhaps a profile—you don’t really need to fetch it through the database anytime. You'll be able to provide it within the cache.
There are 2 common sorts of caching:
1. Server-facet caching (like Redis or Memcached) shops details in memory for quickly obtain.
2. Shopper-aspect caching (like browser caching or CDN caching) suppliers static information near the user.
Caching lessens databases load, improves pace, and will make your app extra effective.
Use caching for things which don’t alter generally. And usually ensure that your cache is updated when knowledge does improve.
In a nutshell, load balancing and caching are very simple but strong tools. Collectively, they assist your application manage additional users, remain rapid, and recover from difficulties. If you propose to grow, you will need both equally.
Use Cloud and Container Tools
To construct scalable purposes, you need resources that allow your application mature effortlessly. That’s in which cloud platforms and containers can be found in. They offer you adaptability, reduce setup time, and make scaling Significantly smoother.
Cloud platforms like Amazon World wide web Products and services (AWS), Google Cloud System (GCP), and Microsoft Azure let you rent servers and expert services as you would like them. You don’t have to purchase hardware or guess potential capability. When site visitors will increase, you may insert extra means with just some clicks or quickly using vehicle-scaling. When traffic drops, you can scale down to save money.
These platforms also supply providers like managed databases, storage, load balancing, and safety resources. You are able to concentrate on developing your app in lieu of running infrastructure.
Containers are A further critical Device. A container deals your app and everything it really should operate—code, libraries, options—into one particular unit. This makes it quick to maneuver your app between environments, from a laptop computer for the cloud, with out surprises. Docker is the preferred Resource for this.
When your application works by using several containers, resources like Kubernetes assist you take care of them. Kubernetes handles deployment, scaling, and recovery. If a single component within your app crashes, it restarts it automatically.
Containers also enable it to be simple to separate portions of your app into products and services. It is possible to update or scale components independently, and that is great for general performance and dependability.
In short, employing cloud and container tools suggests you are able to scale quick, deploy quickly, and Recuperate promptly when issues transpire. If you'd like your application to develop devoid of limits, start off using these equipment early. They help you save time, minimize possibility, and assist you to keep centered on developing, not repairing.
Observe Almost everything
If you don’t check your software, you received’t know when things go Improper. Checking allows you see how your app is doing, location issues early, and make far better selections as your application grows. It’s a vital A part of creating scalable devices.
Get started by tracking fundamental metrics like CPU use, memory, disk House, and response time. These tell you how your servers and providers are undertaking. Instruments like Prometheus, Grafana, Datadog, or New Relic will let you collect and visualize this information.
Don’t just check your servers—check your app way too. Control just how long it will require for people to load internet pages, how frequently faults materialize, and where by they occur. Logging tools like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s taking place inside your code.
Setup alerts for essential difficulties. As an example, Should your response time goes above a Restrict or simply a company goes down, you'll want to get notified straight away. This can help you deal with troubles rapidly, usually prior to customers even notice.
Checking can be valuable once you make alterations. Should you deploy a click here brand new feature and find out a spike in problems or slowdowns, you are able to roll it again in advance of it brings about genuine damage.
As your application grows, site visitors and data maximize. With no monitoring, you’ll miss out on signs of trouble until eventually it’s also late. But with the right instruments in place, you continue to be in control.
To put it briefly, monitoring aids you keep the app reliable and scalable. It’s not almost spotting failures—it’s about comprehension your method and ensuring that it works very well, even under pressure.
Closing Thoughts
Scalability isn’t only for big corporations. Even little applications need a powerful Basis. By developing diligently, optimizing properly, and utilizing the right equipment, you can Create applications that increase effortlessly without having breaking stressed. Start modest, Imagine large, and Create smart.